A Complete Guide to Redis Cache in Express Node JS
This article focuses on exploring some of the most interesting nuances of Redis Caching in NodeJS.
Before we dive into the implementation, let’s understand the concept of caching and how it can help the performance of our application.
Caching
Caching is a process of maintaining processed data in a temporary storage location in turn the same can be accessed quickly for subsequent requests.
This helps us to make our application perform well and serve many requests.
Why should I opt for cache?
The pivotal goal of choosing a cache is to complete the client requests as soon as possible with the help of using a Cache, the data retrieved /processed gets stored in a cache.
The subsequent requests are served from Cache instead of re-retrieve / re-processing. The major role of caching is to speed up the response time of requests sent to the server.
Thereby the cache is the best option for achieving our goal.
There are various ways to handle caching in Node JS but in this article, we are focusing on one of the solutions Redis.
Let's see what Redis is.
Redis
Redis (Remote Dictionary Server) is an open-source and external caching in NodeJS. It provides distributed caching mechanism for cluster-based applications and increases the scalability of the application.
It has a key-value data storage system. It retrieves data in less amount of time compared to Database. Storing and retrieval of data are simple.
See the diagram below to get an idea behind Redis.
Most of the application depends on data processing, in which data comes from a database.
Generally, we send requests and get a response from the server. The same process repeated and again. Therefore, the response time gets increased, and it reduces our app performance.
Here, Redis takes place to rectify the problem and enhance the functionality of our app.
Redis has several methods to access data. For example,
-
set (key, value, option) - responsible for storing data to the key. The option is optional.
-
get(key) - responsible for retrieving data of the key.
Let’s make it work in NodeJS
In the implementation of the Nodejs, we need to do a local configuration setup for Redis.
To use Redis, we must install Redis Server on your local machine and visit the official quickstart guide for getting started.
It is a straightforward approach to installing Redis Server on Linux and Mac. But for Windows, it’s slightly complicated because Redis is officially not supported on Windows.
However, there is a way to install Redis on Windows for development. Read more on How to install Redis on Windows.
After installation, run the command redis-server in the terminal to launch the Redis server, and the screen below will be displayed.
To access the Redis CLI, run the command redis-cli in the terminal and see the following screen:
Once the Redis server is running, start implementing Redis caching in our application.
Create a NodeJS application first and then perform the commands below to install Redis, Express, and Axios.
Let's discuss a detailed explanation of the code above.
npm install redis express axios
Then, use the code below to create a redis client and link it to the redis server.
const redisClient = redis.createClient();
redisClient.connect();
Invariably the application is connected to the local redis server at port 6379 and see the code below to connect to the remote server.
const redisClient = redis.createClient({
url: process.env.REDIS_URL // connection URL of redis
});
In this step, we are going to use an API endpoint (GET/products) which is used to get all the product data from the Fake API (database). Here we are using a Fake API record from dummyjson.com.
app.get("/products", async (req, res) => {
...
});
Whenever a client request (GET/products) is made by the server. It will check for getting data from the Redis store to that specified key(“products”) by using the get(key) method.
const data = await redisClient.get('products');
If the data is not found, it will fetch data from the Fake API (database) and store it into the redis cache using the set (key, value) method and respond to the client.
if(!data) {
const response =
await axios.get('https://dummyjson.com/products');
redisClient.set('products', JSON.stringify(response.data));
res.json(response.data);
}
If the data is found, the result is delivered from the cache to the requesting client.
if(data) {
res.json(JSON.parse(data));
}
Now, let’s test the application.
Open any browser or an API testing platform like postman, swagger, etc., and send the request to the endpoint http://localhost:5000/products.
On this first request, the redis store does not contain data for that key. Therefore, it takes 1.35s to fetch data from the fake API (database).
From the next request onwards, Redis contains data for that particular key. Hence, it takes only 7ms to fetch data from the Redis store.
As you see from the above result, it’s a massive performance improvement of our application.
Conclusion
This blog is quite evident to show you why Redis Cache is used in NodeJS.
We have demonstrated that application performance was accelerated in the implementation which is mentioned above.
Redis is thus a befitting choice for caching.
Want to know more about Redis? Or want to hire nodejs developers for your app development?
We have Nodejs experts who are ready to work on your project. Shall we connect? Contact us.
Recommended Articles:
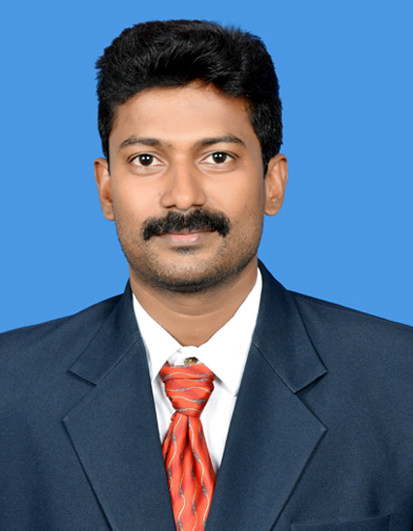
Suresh
I am a Full Stack Developer with over a year of experience, specializing in React, NodeJS, PostgreSQL, and MongoDB. Quick and smart learner is my best attribute.