Redux Middleware
Every React application requires proper state management and Redux continues to dominate in this market. However, if Redux alone is used, a lag can be noticed during asynchronous activities. This is where the Redux middleware enters the picture.
Before proceeding with Redux Middleware, Let’s first have a quick overview of Redux.
Redux
Redux is an open-source JavaScript front-end development library. It is a data flow architecture(state management library) for applications. It is used to overcome state transfer problems between components.
It keeps the state of the whole application in a single immutable store, which cannot be modified directly. When there is any change, a new object of the state is created(using actions and reducers).
Redux Middleware
Redux middleware serves as a bridge between dispatching an action and passing it on to the reducer. Every action that is submitted to the reducer may be intercepted using Redux Middleware, for allowing the user to modify or stop the action.
Why Redux Middleware?
Since Redux is a synchronous process, how does it function with async operations? Middleware for Redux is the solution.
Only synchronous updates are possible if the basic Redux store is used. Redux Middleware is the ideal solution to increase the possibilities of the store.
With the help of middleware, asynchronous requests, error reports, logging, and much more can be made.
To perform asynchronous operations, the actions can also be postponed if needed. Once the asynchronous operation is finished, the rest of the Redux flow proceeds as usual, with the reducer receiving the action to calculate the new state.
Techniques
Several techniques may be utilised for async operations as Redux middleware.
-
Thunk middleware(redux-thunk).
-
Saga middleware(redux-saga).
-
Promise-based middleware.
-
Async/Await (with the help of Thunk middleware).
Let us discuss Thunk Middleware using Redux Toolkit in this blog.
Redux Thunk
Redux Thunk is the most well-known middleware that enables calling action creators and having them return a function rather than an action object. It is used when connecting asynchronously to fetch or store data from an external API.
Prerequisites
To understand Redux Toolkit, one should be familiar with Redux. To learn how to use Redux Toolkit to create Redux apps, refer to my previous blog - Redux in React – A Complete Guide.
Redux-Toolkit includes redux-thunk middleware by default, as thunks are the fundamentally recommended side effect middleware for Redux.
Implementation
Let’s build a simple react application with redux to get users from external fake API. An immersive demo is available on CodeSandbox.
Step 1: Install Dependencies
Install the react-redux and @reduxjs/toolkit libraries in your react app.
npm install react-redux @reduxjs/toolkit |
Step 2: Create Slice function
Create a new file for the slice function, which contains store and reducer used to alter store data.
UsersSlice.js
createSlice API is standard for building Redux logic, in which the reducers object handles synchronous requests to the store and extraReducers handles asynchronous requests.
In the above implementation, fetchUsers is a function that runs an asynchronous operation with the help of createAsyncThunk API.
However, Redux Toolkit allows createAsyncThunk API to delay the asynchronous logic, before passing the processed result to the reducers, and also it accepts three arguments, a string action type value, a callback function(payloadCreator), and an options object which is optional.
The first parameter ‘users/fetchUsers’ is an action type, the second parameter payloadCreator callback which helps to run asynchronous logic and the third parameter is the options parameter which is an object that contains several settings for the createAsyncThunk API. Examine the alternatives offered.
Whenever the fetchUsers function is dispatched from a component, createAsyncThunk produces promise lifecycle action types with this string as a prefix.
-
pending: users/fetchUsers/pending
-
fulfilled: user/fetchUsers/fulfilled
-
rejected: user/fetchUsers/rejected
users/fetchUsers/pending lifecycle action type gets dispatched on its initial call. Then, the payloadCreator runs to either return data or an error.
users/fetchUsers/fulfilled lifecycle action type gets dispatched when the data fetch is successful.
user/fetchUsers/rejected lifecycle action type gets dispatched when there is a failure while fetching the data.
The above-described three lifecycle action types can be assessed in the extraReducers object, wherein we perform the store modifications that are required. Let's assign users with data and set the loading status for each action type suitably.
Step 3: Configure Redux Store in Application
Import the following -
-
configureStore from ‘@reduxjs/toolkit’ and
-
Provider from ‘react-redux’
in the top-level component, for connecting the usersReducer to the application.
App.js
The middleware option can also be used in configureStore API to customise the middleware.
All middleware that is required to be added to the store must be defined. Beyond what is provided, configureStore will not install any further middleware.
For example,
const store = configureStore({ |
If some custom middleware or third-party middleware is needed, but don’t want the default thunk middleware, in this case, getDefaultMiddleware is helpful:
For example,
const store = configureStore({ |
Step 4: Utilize Redux in the component using hooks
Let’s create a Users Component to display the users name.
In the above component, we can read the state values from a Redux store and dispatch actions from a component using the useSelector and useDispatch functions in the react-redux library.
Conclusion
In this blog, we have extensive discussions about redux middleware. If anyone is new to Redux, it is recommended to start with Redux Thunk, which is simple to learn and apply. Once mastered Thunks, driving onto promise-based middleware and Saga’s are more beneficial.
Further, Redux-Saga is a feature-rich and ideal solution for big applications that require scaling. Since it handles application side effects effectively, is straightforward to test, and makes handling errors easier.
Do you have queries about Redux? Or do you want to connect with React developers?
Infinijith will provide customized solutions to your needs.
Talk to our developers!
Recommended Article:
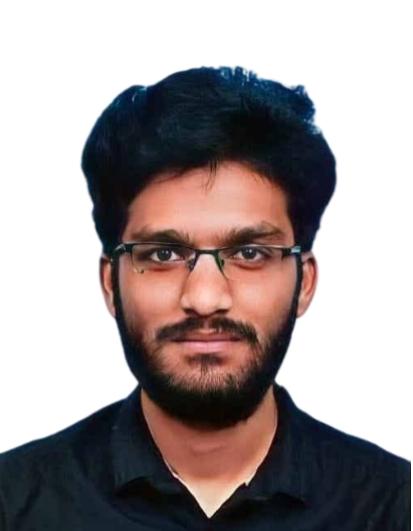
Vasanth
I am a skilled full stack developer with expertise in front-end and back-end development. React, Angular, Vue, NextJS, NuxtJS, Nodejs, DotNet, MySQL, Postgres, SQL Server, MongoDB.