Cross-Origin Resource Sharing - How to Solve CORS Errors
All the developers must have faced the CORS error when trying to make a request from the client to the server.
For example,
When we try to fetch data on the origin http://localhost:4000 from the server located at http://localhost:3000, it responds with a weird error as shown in the picture below.
If you do not know how to resolve this technical glitch, you are on the right page. In turn, you can learn the overall concept of CORS to fix CORS errors.
Let's start with CORS Concept
CORS stands for Cross-Origin resource sharing. Simultaneously, it helps modern browsers to perform cross-domain resource access, but what does it mean?
It is a browser mechanism in which, the clients run at one origin to access server resources at another origin via CORS HTTP headers.
Why CORS is necessary for us?
-
To prevent unauthorized domains from accessing data on servers.
-
It is excessively used for data security and authorized communication between clients and servers.
CORS was established to make simple and quick-to-reach subdomains and reputable third parties. Before we get into the CORS examples and error fixing, we have to know the other concept known as the Same-Origin Policy.
The Same-Origin Policy (SOP)
The Same-Origin Policy permits data sharing between clients and servers with the same origin.
Let’s see we have a client with the origin http://localhost:3000. Then we make a request to a server with an endpoint http://localhost:3000/products that will be allowed because both are under the same origin.
Client-side CORS
By default, we can fetch resources from the server using the same origin. However, the browser forbids us from accessing resources that aren't at the same origin. In such a case, the browser utilizes a mechanism known as CORS to safely permit cross-origin requests.
Server-side CORS
In the process of a backend developer, we must ensure that cross-origin requests are approved by adding extra headers to the HTTP response. Based on the response headers, the browser can allow the cross-origin response.
"You must ensure that your server sends the valid response headers to handle a CORS issue."
There are several headers available to configure CORS, which covers all with Access-Control-*.
This article throws light on the Access-Control-Allow-Origin and Access-Control-Allow-Methods Headers.
Let’s discuss the Access-Control-Allow-Origin header.
The Access-Control-Allow-Origin header enables Cross-Origin resource sharing.
For example, if you want to make a request between the client and server in different Domains/URLs. Then you need to make the ‘Access-Control-Allow-Origin’ header from server to client on each request.
To allow all domains use the wildcard (*) as value:
Access-Control-Allow-Origin: *
To allow certain domains use the domain URL:
Access-Control-Allow-Origin: “http://localhost:4000”
If we create a server that should have access to http://localhost:4000, then we can add the value of that domain to the Access-Control-Allow-Origin header.
For example,
response.setHeader("Access-Control-Allow-Origin", "http://localhost:4000")
The CORS mechanism in the browser verifies that the Origin sent with the request matches the value of the Access-Control-Allow-Origin header in the server.
The response that the server now sends back successfully to the client includes these cross-origin headers and the data.
Now, let’s discuss the Access-Control-Allow-Methods header.
The Access-Control-Allow-Methods is another header. CORS will accept requests that were sent using one of the methods listed in the header.
For example,
response.setHeader( "Access-Control-Allow-Methods", ["GET", "POST"])
In the afore-mentioned example, only requests with GET, and POST methods will be allowed. Other methods such as PUT, PATCH, or DELETE will be blocked by CORS.
CORS Request Types
CORS has different types of requests:
-
Simple request
-
Preflighted request
Simple Request
When the request is a GET or POST, HEAD method and doesn’t have any custom headers that are called a Simple Request.
A simple request needs to satisfy each of the requirements listed in this link.
CORS Preflight request
When the PUT, DELETE, PATCH, or other complex request or
simple request (GET, POST) with any custom headers is made, the browser by default starts a Preflighted request (with the OPTIONS HTTP method) to the server to determine whether the actual request can be processed or not. This preflight request contains Access-Control-Request-Method, Access-Control-Request-Headers, and Origin headers.
For Example,
When you make a PUT request to a server, The browser sends a preflight request (OPTIONS) to the server.
After receiving this preflighted request, the server receives a reply with an empty HTTP response along with the CORS headers. The browser gets the response and determines whether to allow or not to allow the actual PUT request.
If allowed, the browser sends the actual request to the server and the server responds with the requested data.
Conclusion
This blog has given you a vivid and conceptual understanding of CORS. Therefore, I hope you will be able to solve the CORS errors.
If you need developers for your project, feel free to contact us.
Get a free quote and talk to our developers to start your project.
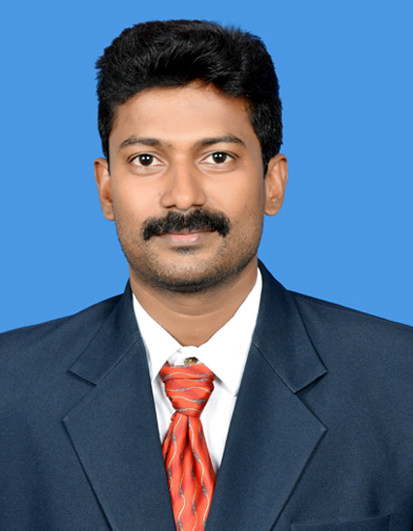
Suresh
I am a Full Stack Developer with over a year of experience, specializing in React, NodeJS, PostgreSQL, and MongoDB. Quick and smart learner is my best attribute.