React Hooks - A Complete Guide For 2023 (Part – 1)
React Hooks
React Hooks are functions that allow you to access state and life-cycle methods in function components without using class components.
Hooks are introduced as a brand-new feature in React16.8. Before React 16.8, we had been utilizing class components such as stateful components, functional components and stateless components which mean we were unable to use state in the functional components.
However, thanks to hooks, we are now able to integrate state, lifecycle methods, and other class-based features in functional components. Most importantly, Hooks cannot be used in class components.
Before we investigate the hooks, let's go through the best practices to use hooks:
-
Use hooks only at the top level.
-
Always call hooks from React function Component.
-
Hooks can't be called inside the conditions, loops, nested functions and in regular javascript functions.
Types of Hooks
React has several built-in hooks, which are divided into two pieces, they are shown below.
Basic hooks
-
useState
-
useEffect
-
useContext
Additional Hooks
-
useReducer
-
useCallback
-
useMemo
-
useRef
In this article, the paramount focus is on the basic hooks of React.
State Hook
useState Hook is a method for declaring a state in a React app. It helps to set and retrieve the state (value) and re-renders the component whenever there is a state change.
It is declared like
const [state, setState] = useState(initialValue);
As you see in the above syntax for useState, it takes one argument as an initial value and returns an array of two values
-
First Value (state) - It is a variable with the current value.
-
Second Value (setState) - It is a method to update the current value and re-render the component.
Let us take a quick example, first import the useState hook from React Library and use it
import { useState } from "react";
In the above example, initially the count value is zero. Whenever we click the increment button, the previous value of the count will be incremented by 1 and with updation the count value increases using the setCount() method.
Note: When we trigger the setCount() method, the component will re-render.
Effect Hook
useEffect hook is used in functional components to allow us to perform some logic (side effect) after every render of the component. It is the close replacement of the class component lifecycle methods such as
-
componentDidMount
-
componentDidUpdate
-
componentWillUnmount.
It is declared like
useEffect(callback(), [dependencies]);
As you see in the above syntax for useEffect, it takes two arguments.
-
Callback () - it is a callback function to handle and clean upside effects.
-
[dependencies] - it is a dependency array to run a callback function when a dependency changes.
useEffect allows us to do various activities smoothly. The examples include
-
Fetching and consuming data from a server API.
-
Managing subscriptions using event listeners and timers.
-
Updating the DOM and so on.
Now, let us plunge into the useEffect Concept. In this article, I covered 6 ways to use the useEffect() method.
First, you need to import the useEffect hook from the React Library.
import { useEffect } from "react";
Runs after every render:
This case executes the callback function on every render of the component when we do not pass the second parameter (dependency array). This is the default case.
useEffect(() => {
// doSomething
});
Runs only once after the initial render:
In this case, the callback function executes only once after the initial rendering of the component. It is mostly used when making an API call to fetch data and store it to the state after the initial rendering.
useEffect(() => {
// doSomething
}, []);
For this use, in case we need to send an empty array as the second parameter.
Runs after State value changes:
This case executes the callback function when the state value changes. For this use case, send the state variable to the dependency array.
useEffect(() => {
// doSomething
}, [state]);
This is also applicable for multiple state variables. For that, send the state variable to the dependency array as comma-separated values.
useEffect(() => {
// doSomething
}, [state1, state2]);
Runs after the Props value changes:
This is also the same as the above one, but the callback function executes when the props value changes. For this use case, send the props variable to the dependency array.
useEffect(() => {
// doSomething
}, [props]);
Runs after Props and State value changes:
This is the combination of State and Props. The callback function executes when the props or state value changes. To address this use case, send both the state and props variable to the dependency array as comma separated.
useEffect(() => {
// doSomething
}, [state, props]);
Runs before component destroys (Effect Cleanup):
We know when and how to execute a side effect. In addition to focusing on the performance of the application, we must clean up the side effects.
To use the clean-up function, you need to include the return function from the callback function.
This case runs the clean-up function before the component gets unmounted from the UI to prevent data breaches and removes unwanted behaviors.
These are the main side effects that clean up when the component unmounts.
-
Timers.
-
Event listeners.
-
Subscriptions.
-
Effects that are no longer needed.
useEffect(() => {
// doSomething
return () => {
// Cleanup
}
}, [state, props])
Context Hook
If you are a React developer, you probably know that everything in react is a component and passed props from the parent to the child component.
However, if your application expands, this can become difficult and impractical to share props between many components.
To overcome that problem the Context hook helps us to save the application's state globally and share it among several components. The hook which helps to solve this problem is called the useContext hook.
It is declared like
const value = useContext(SomeContextName)
As you see in the above syntax for useContext, it takes one argument which is the Context object, and returns the context value for the context passed.
Okay, let's examine an example to grasp this better.
Firstly, you need to import the createContext from React Library and initialize it.
import { createContext } from "react";
const TestContext = createContext();
Then it will use the Context Provider to wrap the hierarchy of components that need the state value by using the TestContext.Provider along with some value prop to it.
The TestContext provider is set with a value of “test”. Now, all the child components (ChildComponent) wrapped inside the context provider will be able to use the value.
To use that value, we are going to use the useContext hook. Let us see in the code.
Intially, Import the useContext hook from the react library and use it in component
import { useContext } from 'react';
Above all, the component that uses useContext will always be re-rendered when the context value changes.
Conclusion
Hooks helped us to improve our code design and app performance and it is recommended to you to utilize them in your projects as well.
That is all about the usage of the basic hooks, I hope this article is informative, educational, and useful.
In the next article, we will discuss the additional hook types and continue to dive into React's great and extremely cool features.
Check that article here: React Additional Hooks
P.S: If you are looking to hire react js developers or react native developers, add us to your search list.
Being a full stack development company, Infinijith provides excellent reactjs app development services.
Talk to our react experts to work together!
Recommended Articles:
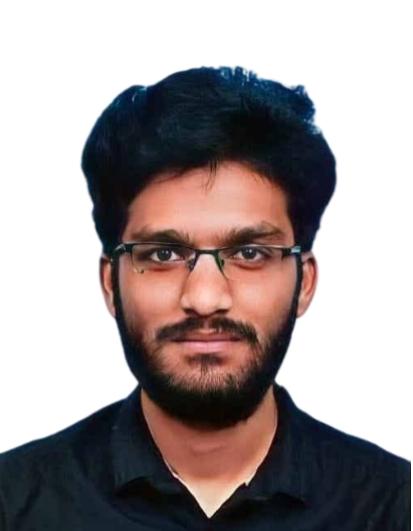
Vasanth
I am a skilled full stack developer with expertise in front-end and back-end development. React, Angular, Vue, NextJS, NuxtJS, Nodejs, DotNet, MySQL, Postgres, SQL Server, MongoDB.