React Hooks - A Complete Guide For 2023 (Part – 2)
The React hooks are instrumental in the functions that enable access to the functional component’s state and life-cycle characteristics. If you have experienced React, you must have probably used the built-in Hooks like useState, useEffect, and useContext.
Note: If you are unfamiliar with basic hooks, read the earlier blog here: React basic hooks, where we discussed what they are, recommended practices, types and detailed explanation of basic hooks. Before beginning this post, make sure you read them.
In this article, we will explore the operations of various additional hooks of react and learn how to use them.
Additional Hooks
-
useReducer
-
useRef
-
useMemo
-
useCallback
useReducer Hook
useReducer is equivalent to useState. It is often chosen when the next state depends on the previous one or when you have complicated the state logic involving several values.
It is declared as follows:
const [state, dispatch] = useState(reducer, initialValue);
As you see in the above syntax for useReducer, which takes action and the initial state and returns the current state along with a dispatch mechanism.
Let us take an example, first import the useReducer hook from React Library and use it
import { useReducer } from "react";
Initially, the count is 0. When we click on the Increment button, it triggers the dispatch function; in turn it sets the value of the action to type ‘increment’ and runs the reducer function.
The reducer function executes the block of code and checks the action type ‘increment’ in the switch case. Once the type matches, it returns an incremented value to the count. Likewise, when we click the Reset button, this action triggers the dispatch function and checks the type ‘reset’ in the reducer function and returns 0 to the count.
useRef Hook
useRef enables you to directly create a reference to the DOM element in functional components. It is also capable of holding a mutable value in its current attribute. It is used to align with the useState hook when the value is rapidly changing. However, it does not require a re-rendering of the value when it changes.
It is declared like
const ref = useRef(initialValue);
As you see in the above syntax for useRef, it takes one argument as primary Value and returns the reference of the DOM element in its current property.
First, you need to import the useRef hook from React Library.
import { useRef } from "react";
In the above example, we set the state of inputRef to the input element using the ref attribute. Now, when we click the button, the inputRef variable's current value is used to place the focus on the input element.
useMemo Hook
useMemo hook is a memorization function that catches a value returned by an expensive function and improves performance. The expensive function should only be called on the initial render or when a referenced dependency value has changed, according to the optimization idea.
It is declared like
const memoizedValue = useMemo(callback(), [dependencies]);
As you see in the above syntax for useMemo, it takes two arguments and returns an expensive value.
-
callback() - it is a callback function to handle the expensive task and the return value.
-
[dependencies] - it is a dependency array to run a callback function when the dependency changes.
To start with, you need to import the useMemo from React Library.
import { useMemo } from "react";
First, let us discuss the above example without useMemo. Whenever we click the increment button, the function increments the count value using the setCount method and re-renders the component. Subsequently, Child Component also re-renders whenever Parent Component renders. This will reduce our app performance.
However, Let us have a look at, how useMemo takes place to avoid that. In this circumstance, useMemo can provide us with a memoized output by simply wrapping the child component with the useMemo to call ChildComponent.
const childComponent = useMemo(() => <ChildComponent />, []);
At this juncture, when we click the increment button, the function increments the count value using the setCount method and re-renders the component. However, ChildComponent will not re-render when ParentComponent renders.
useCallback Hook
useCallback hook is also a memorization function that returns a cached version of a function to improve performance.
When a component is re-rendered the entire function inside a component and regenerated, thereby the references to these functions vary between renderings.
In such case, useCallback returns a memoized function of the callback that changes only if one of the dependencies changes. This implies that we may reuse the same function object between renderings rather than constructing a new one for each render.
It is declared like
const memoizedFunction = useCallback(callback(),[dependencies]);
As you see in the above syntax for useCallback, it takes two arguments and returns a memorized function.
-
callback() - it is a callback function to handle expensive task and returns the cached callback function.
-
[dependencies] - it is a dependency array to run a callback function when a dependency changes.
To begin with, you need to import the useCallback from React Library.
import { useCallback } from "react";
In the above example, there are 2 components they are as follows:
The app and the button. In the App component, we have 2 states - count1 and count2 and 2 functions -incrementCount1 which increments the count1 by 1, and incrementCount2 which increments the count 2. In the Button component, we have a button that handles operations based on problems from the App component.
Let us see the above example without useCallback, when we click any of the increment buttons, it sets the incremented count value and re-renders all the components. However, it is also recreated at all the functions again.
To overcome that, simply wrapping those functions inside the useCallback hook helps us to cache those functions and return them only when dependencies change. In the example, we wrapped the Button component by memo method to prevent unnecessary re-renderings.
const incrementCount1 = useCallback(() => setCount1(count1 + 1), [count1]);
const incrementCount2 = useCallback(() => setCount2(count2 + 1), [count2]);
Now, when we click any of the increment buttons, it sets the incremented count value and re-renders only the memoized function component and does not render other components.
Conclusion
Additional hooks in React are extensively used to improve the performance of the app. I hope this article explains to you vividly to understand the primary additional hooks.
Are you in search of React developers? Hire reactjs developers at Infinijith for your upcoming project and start developing your app.
Get your free quote now!
Recommended Articles:
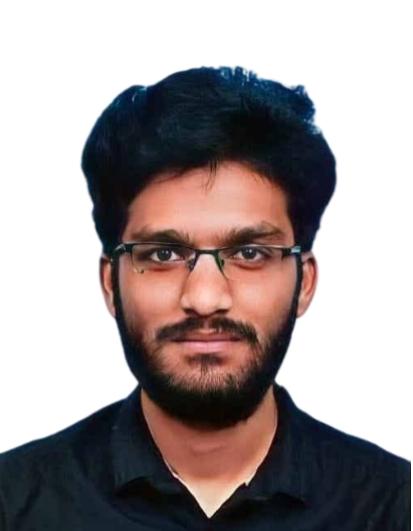
Vasanth
I am a skilled full stack developer with expertise in front-end and back-end development. React, Angular, Vue, NextJS, NuxtJS, Nodejs, DotNet, MySQL, Postgres, SQL Server, MongoDB.